Table Of Content
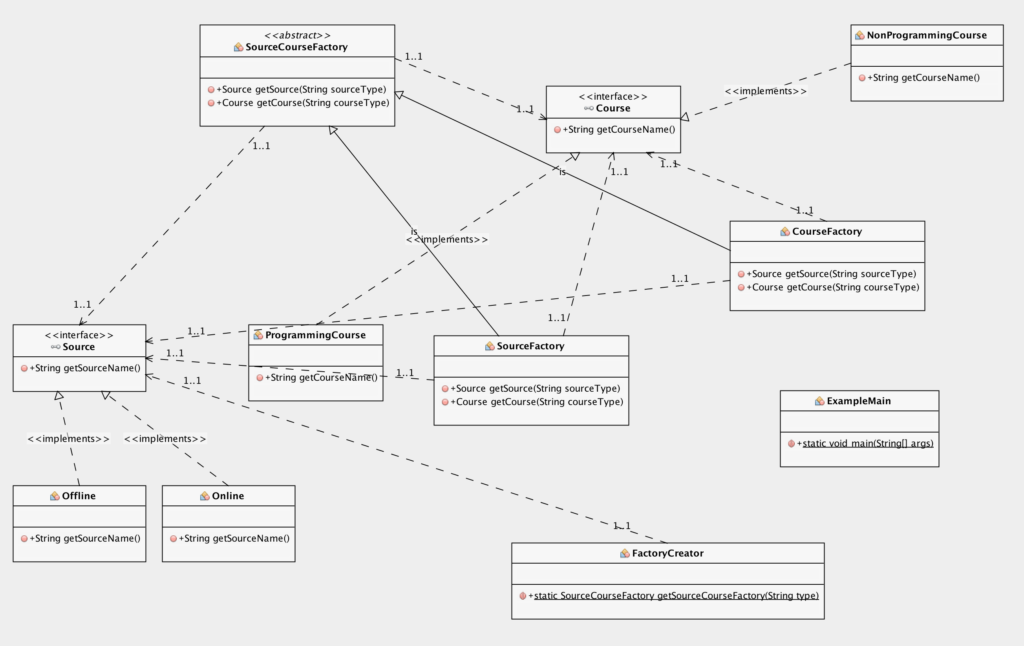
When the factory method comes into play, you don’t need to rewrite the logic of the Dialog class for each operating system. If we declare a factory method that produces buttons inside the base Dialog class, we can later create a subclass that returns Windows-styled buttons from the factory method. The subclass then inherits most of the code from the base class, but, thanks to the factory method, can render Windows-looking buttons on the screen. The abstract creator class, ShapeFactory, declare a pure virtual function createShape(), which will be implemented by concrete creators to create specific shapes. Concrete Product classes are the actual objects that the factory method creates.
Factory Design Pattern
Classes can provide additional interfaces to add functionality, and they can be derived to customize behavior. Unless you have a very basic creator that will never change in the future, you want to implement it as a class and not a function. A client (SongSerializer.serialize()) depends on a concrete implementation of an interface. It requests the implementation from a creator component (get_serializer()) using some sort of identifier (format). Then, you provide a separate component that decides the concrete implementation to use based on the specified format.
The All-New 2023 Chevrolet Colorado Takes Midsize Trucks to the Next Level - Chevrolet Pressroom
The All-New 2023 Chevrolet Colorado Takes Midsize Trucks to the Next Level.
Posted: Thu, 28 Jul 2022 07:00:00 GMT [source]
Support our free website and own the eBook!
This example is short and simplified, but it still has a lot of complexity. There are three logical or execution paths depending on the value of the format parameter. This may not seem like a big deal, and you’ve probably seen code with more complexity than this, but the above example is still pretty hard to maintain. Adding a new class to the program isn’t that simple if the rest of the code is already coupled to existing classes.
Creational Software Design Patterns in C++
The framework receives the shape type as a string parameter, it asks the factory to create a new shape sending the parameter received from menu. The factory creates a new circle and returns it to the framework, casted to an abstract shape. Then the framework uses the object as casted to the abstract class without being aware of the concrete object type. Room is the base class for a final product (MagicRoom or OrdinaryRoom).
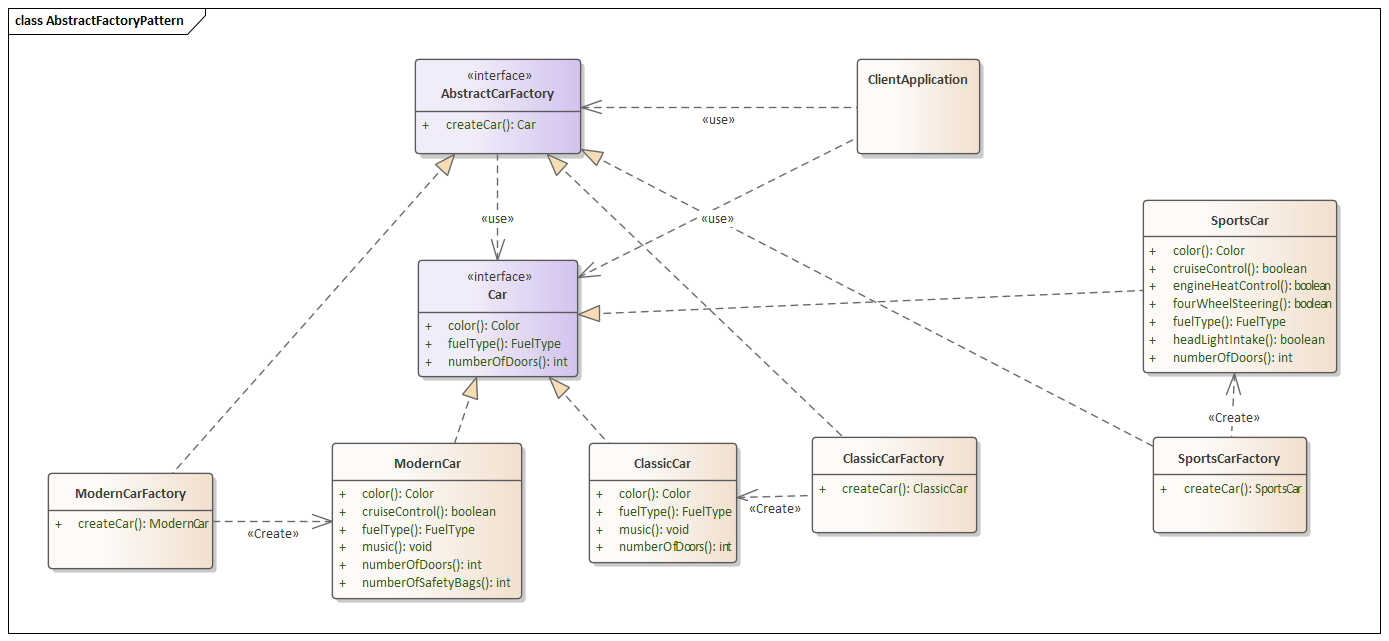
FactoryPatternDemo, our demo class will use ShapeFactory to get a Shape object. It will pass information (CIRCLE / RECTANGLE / SQUARE) to ShapeFactory to get the type of object it needs. However, this would make the code too dependent on each specific change and hard to switch between them easily. The procedural implementation is the classical bad example for the Open-Close Principle. As we can see there the most intuitive solution to avoid modifying the Factory class is to extend it.
Connected Factory Solution based on AWS IoT for Industry 4.0 success Amazon Web Services - AWS Blog
Connected Factory Solution based on AWS IoT for Industry 4.0 success Amazon Web Services.
Posted: Thu, 09 Jul 2020 07:00:00 GMT [source]
As a result, you will end up with pretty nasty code, riddled with conditionals that switch the app’s behavior depending on the class of transportation objects. The first version of your app can only handle transportation by trucks, so the bulk of your code lives inside the Truck class. Design patterns differ by their complexity, level ofdetail and scale of applicability. In addition,they can be categorized by their intentand divided into three groups.
Factory Method as an Object Factory
The creation of each concrete music service has its own set of requirements. This means a common initialization interface for each service implementation is not possible or recommended. The service returns an access code that should be used on any further communication. You can see the basic interface of SerializerFactory in the implementation of ObjectSerializer.serialize(). The method uses factory.get_serializer(format) to retrieve the serializer from the object factory. So far, we’ve seen the implementation of the client (ObjectSerializer) and the product (serializer).
The service requires that the the location of the music collection in the local system be specified. Creating a new service instance is done very quickly, so a new instance can be created every time the user wants to access the music collection. To understand the complexities of a general purpose solution, let’s take a look at a different problem.
Structural Software Design Patterns in C++
For example, your application might require in the future to convert the Song object to a binary format. This interface is implemented by the concrete classes JsonSerializer and XmlSerializer. Pentalog is a digital services platform dedicated to helping companies access world-class software engineering and product talent. With a global workforce spanning 16 locations, our staffing solutions and digital services power client success.
The factory design pattern offers a solution for efficient object creation in software development. It reduces coupling between client code and object construction code and results in maintainable, scalable applications. Understanding when and how to apply the factory design pattern effectively can enhance your skills as a software developer and improve your applications. The Factory Design Pattern is a powerful technique for flexible object creation. By encapsulating the creation logic in a factory class, we achieve loose coupling and enhance the maintainability of our code.
The registration information is stored in the _creators dictionary. The .get_serializer() method retrieves the registered creator and creates the desired object. If the requested format has not been registered, then ValueError is raised. The Song class implements the Serializable interface by providing a .serialize(serializer) method.
Instead of knowing the exact object class and instantiating it through a constructor, the responsibility of creating an object is moved away from the client. So, we have created three Product classes that implement the CreditCard interface. Next, we must consume the Product classes inside the client code by creating and initializing the appropriate Product class object.
Subclassing it means replacing all the factory class references everywhere through the code. These patterns control the way we define and design the objects, as well as how we instantiate them. Finally, the application implements the concept of a local music service where the music collection is stored locally.
Wikipedia has a good catalog of design patterns with links to pages for the most common and useful patterns. Also, Spotify and Pandora require an authorization process before the service instance can be created. The implementation of SerializerFactory is a huge improvement from the original example. It provides great flexibility to support new formats and avoids modifying existing code. By implementing Factory Method using an Object Factory and providing a registration interface, you are able to support new formats without changing any of the existing application code. This minimizes the risk of breaking existing features or introducing subtle bugs.
Finally, we call the draw() method on these objects, which produces the expected output. Client Code is nothing but the class from where we need to consume the product classes (MoneyBack, Titanium, and Platinum). And in our example, it will be the Main method of the Program class. We will ask the user to select the Credit Card Type in the client code. The application defines a config dictionary representing the application configuration.
No comments:
Post a Comment